Mastering iOS Interview Questions: A Comprehensive Guide to Ace Your Interview
Preparing for an iOS interview requires a deep understanding of the intricacies of iOS development and the ability to articulate your knowledge effectively. To help you succeed, we have compiled a comprehensive list of top iOS interview questions along with guidelines to help you answer them confidently. By familiarizing yourself with these questions and following the provided guidelines, you’ll be well-prepared to impress your interviewers and increase your chances of landing your dream iOS developer job.
Read More : PHP Interview Questions
1- Swift Programming Language:
a. What is Swift, and how is it different from Objective-C?
Guideline: Explain that Swift is a powerful and modern programming language developed by Apple for iOS, macOS, watchOS, and tvOS app development. Highlight its advantages over Objective-C, such as its safety, simplicity, and interoperability with Objective-C code.
b. Explain value types and reference types in Swift.
Guideline: Describe that value types, such as structs and Enums, are passed by value, while reference types, such as classes, are passed by reference. Emphasize the implications of this difference, such as value types being copied when assigned to a new variable and reference types sharing the same instance.
c. What are optionals in Swift, and why are they useful?
Guideline: Define optionals as a feature in Swift that allows variables to have a value or be nil. Explain their usefulness in handling situations where a value may be absent. Discuss techniques for safely unwrapping optionals, such as optional binding and optional chaining.
d. Discuss the differences between a structure and a class in Swift.
Guideline: Highlight that both structs and classes can have properties, methods, and initializers, but structs are value types, while classes are reference types. Point out that structs are passed by value, while classes are passed by reference. Emphasize that classes support inheritance but structs do not.
e. What is a closure in Swift, and how is it used?
Guideline: Define closures as self-contained blocks of functionality that can be passed around and used in code. Explain their applications, such as handling asynchronous tasks, sorting collections, and encapsulating functionality. Provide examples of closure syntax and demonstrate how to capture values in closures.
2- iOS Frameworks and Libraries:
a. Describe the purpose and usage of UIKit and Foundation frameworks.
Guideline: Mention that UIKit is the primary framework for building iOS user interfaces, while Foundation provides fundamental classes and utilities. Discuss key components of UIKit, such as UIView, UIViewController, and UIApplication. Explain that Foundation offers classes for data types, networking, file management, and more.
b. What is Core Data, and how is it used for data persistence in iOS apps?
Guideline: Explain that Core Data is a framework for managing the model layer objects in an iOS app. Discuss its features, such as object graph management, data modeling, and support for various persistent stores. Mention that Core Data simplifies data persistence and provides powerful querying capabilities.
c. Explain the role of Core Animation in iOS app development.
Guideline: Describe Core Animation as a graphics rendering and animation framework. Discuss its capabilities, such as animating views, applying transformations, and creating complex animations. Emphasize that Core Animation is used for creating visually appealing and interactive user interfaces.
d. What is the purpose of Core Location framework, and how is it used?
Guideline: Mention that Core Location framework provides services for determining the device’s geographic location, altitude, and heading. Explain its applications, such as location-based services, navigation, and geofencing. Discuss the usage of CLLocationManager and its delegate methods for obtaining location data.
e. Discuss the significance of Alamofire and URLSession in networking tasks.
Guideline: Explain that Alamofire is a popular third-party networking library for iOS, built on top of URLSession. Discuss its advantages, such as simplified API, automatic request and response serialization, and network reachability checks. Explain that URLSession is Apple’s native networking framework, providing powerful networking capabilities.
3- App Architecture and Design Patterns:
a. What is the Model-View-Controller (MVC) design pattern, and how is it used in iOS development?
Guideline: Explain that MVC is a design pattern that separates an app into three components: model, view, and controller. Describe the responsibilities of each component, such as managing data, displaying the user interface, and handling user interactions. Emphasize the importance of adhering to MVC principles for code organization and maintainability.
b. Explain the concept of delegation and provide an example of its usage in iOS.
Guideline: Describe delegation as a design pattern where one object delegates tasks or responsibilities to another object. Provide an example, such as the UITableViewDelegate and UITableViewDataSource protocols used for configuring and managing a UITableView. Discuss how delegation promotes loose coupling and modular design.
c. What is the difference between notifications and KVO (Key-Value Observing) in iOS?
Guideline: Explain that notifications allow objects to broadcast information to other objects, while KVO enables objects to observe changes to specific properties of other objects. Discuss that notifications use the Notification Center to publish and receive information, while KVO relies on observing changes using add Observer(_:forKeyPath:options:context:). Highlight that notifications are more suitable for broadcasting events, while KVO is ideal for observing state changes.
d. Discuss the benefits and implementation of the Singleton design pattern.
Guideline: Explain that the Singleton design pattern ensures a class has only one instance globally accessible. Discuss its benefits, such as providing a shared resource, centralized access, and lazy initialization. Demonstrate the implementation of a Singleton class using a static shared instance and private initializer.
e. What is the purpose of the coordinator design pattern in iOS app navigation?
Guideline: Describe the Coordinator design pattern to separate navigation logic from view controllers. Explain that coordinators manage the flow of screens and handle transitions between them. Discuss the benefits of using coordinators, such as improved testability, modularity, and easier navigation management.
Read More : python developer job description
4- User Interface (UI) Development:
a. Describe Auto Layout and its role in creating adaptive user interfaces.
Guideline: Explain that Auto Layout is a constraint-based layout system that allows building adaptive user interfaces. Discuss how Auto Layout ensures views adapt to different screen sizes, orientations, and accessibility settings. Highlight the use of constraints to define the relationships between views.
b. What are the different types of constraints in Auto Layout, and how are they prioritized?
Guideline: Explain the different types of constraints, such as equality constraints, inequality constraints, and content-hugging and compression-resistance priorities. Discuss how Auto Layout uses priority levels to resolve conflicting constraints, ensuring the appropriate layout.
c. Explain the purpose and usage of UITableView and UICollectionView.
Guideline: Describe UITableView as a view for presenting structured, scrolling lists of data, and UICollectionView as a more flexible view for creating custom layouts of items. Discuss their common usage patterns, such as data source and delegate implementations, cell registration, and handling user interactions.
d. How can you handle user input using gestures in iOS apps?
Guideline: Explain that gestures allow users to interact with iOS apps through touch-based input. Discuss common gestures, such as tap, swipe, pinch, and rotation. Explain how to handle gestures using gesture recognizers and their associated action methods.
e. Discuss the benefits and usage of UIStackView in UI layout management.
Guideline: Explain that UIStackView is a container view that simplifies the process of laying out a collection of views in a stack-like arrangement. Discuss its benefits, such as automatic layout, easy distribution and alignment, and dynamic content handling. Demonstrate how to use UIStackView in Interface Builder or programmatically.
5- App Lifecycle and Persistence:
a. Explain the different states of an iOS app during its lifecycle.
Guideline: Discuss the app lifecycle states, including not running, inactive, active, background, and suspended. Explain the transitions between states and the events triggered during each phase. Emphasize the importance of handling these lifecycle events for managing resources and maintaining a responsive user experience.
b. What is the purpose of the AppDelegate class, and what methods are commonly used?
Guideline: Explain that the AppDelegate class is the entry point and central coordinator of an iOS app. Discuss commonly used methods, such as application(:didFinishLaunchingWithOptions:), applicationDidBecomeActive(:), applicationWillResignActive(:), and applicationDidEnterBackground(:). Highlight their significance in managing app initialization, state changes, and background tasks.
c. How can you save and restore the state of an iOS app when it is terminated or backgrounded?
Guideline: Discuss techniques for saving and restoring app state, such as using UserDefaults to store simple data, implementing state restoration in the AppDelegate, and leveraging persistence frameworks like Core Data or Realm for complex data structures. Emphasize the importance of data serialization and error handling.
d. Discuss the options for data persistence in iOS apps, including UserDefaults and Core Data.
Guideline: Explain that User Defaults is a lightweight option for storing small amounts of data, such as user preferences and settings. Discuss Core Data as a more robust framework for managing complex data models and providing advanced querying capabilities. Highlight the trade-offs and considerations when choosing between these options.
e. Explain the purpose and implementation of background tasks and multitasking in iOS.
Guideline: Discuss the purpose of background tasks, such as performing network requests, updating content, or handling location updates while the app is in the background. Explain how to implement background tasks using background modes, background fetch, background notifications, and background URL Session configurations.
6- Testing and Debugging:
a. What is unit testing, and how can you perform unit testing in iOS apps?
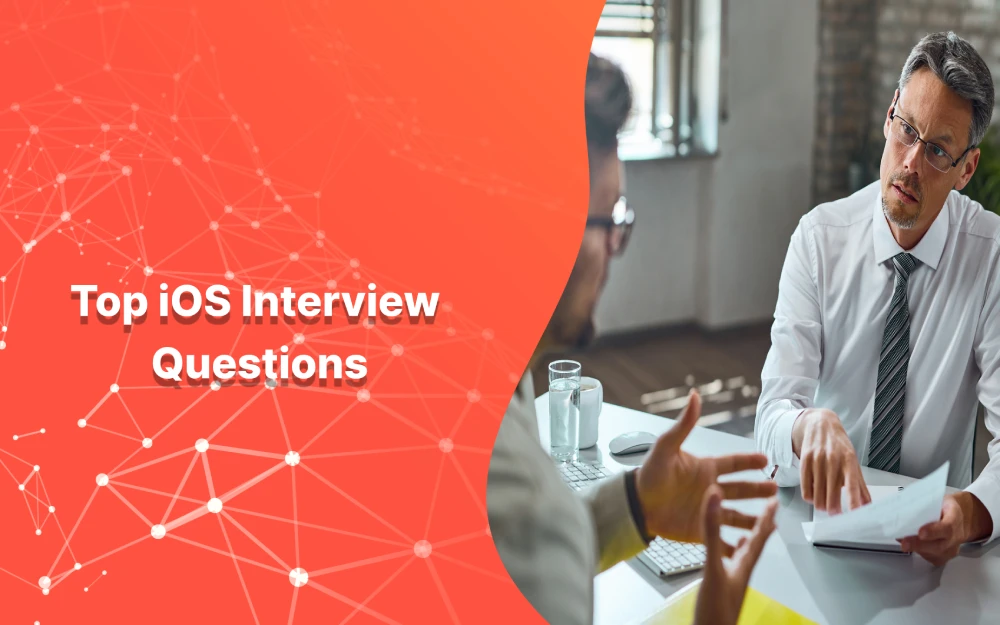
Guideline: Define unit testing as the practice of testing individual units of code to ensure their correctness. Discuss XCTest, Apple’s built-in testing framework, and explain how to write test cases, assertions, and setup/teardown methods. Emphasize the importance of writing testable code and running tests regularly.
b. Discuss the benefits and usage of Xcode’s debugging tools.
Guideline
In Techitopia, it will help you more in overcoming this type of stress in interviews by giving all the advice and information to be fully qualified to overcome any type of interview.